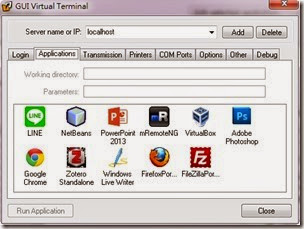
這篇記錄一下最近研究RemoteApp的記事。由於不是很正式的教學,所以格式上比較隨意,請大家見諒。
This article is the memo about RemoteApp research.
為什麼個人要用RemoteApp? / Why I Need RemoteApp?
在講RemoteApp之前,我先講一下什麼樣的情況,個人會須要用到這種方案。
現代人的生活很多時候會跟多臺電腦打交道。以我為例,先不論機房有一堆孩子(伺服器)要顧,我自己在實驗室有一臺桌機、平時在外面移動時則是有一臺筆電,回到宿舍時也還是用那一臺筆電工作。因此我自己就有兩臺電腦在使用。
在這個情景底下,我主要使用的是實驗室效能比較好的桌機,而筆電通常是利用遠端桌面連線回到桌機操作。大部分的檔案也是存放在桌機為主。
Windows的遠端桌面連線是Windows少數幾個偉大的設計之一。在網路頻寬允許的情況下,可以讓你的連線裝置彷彿就像是在遠端操作一樣。
但是實際上,遠端桌面連線因為功能太過龐大,有時候我只是想要使用某個應用程式,像是Photoshop,這時候我還是得連線到整個遠端桌面,然後才能開啟Photoshop操作,說來也是一種麻煩。
這就讓我興起了「我可只連線到遠端其中一個應用程式嗎?」的想法,因此就開始進行RemoteApp的小小研究。
遠端桌面連線的RemoteApp / RemoteApp of Remote Desktop Service
這篇所講的RemoteApp,一開始是指Windows伺服器版本提供的功能。RemoteApp是遠端桌面連線的延伸應用,自從Windows Server 2008 R2之後才能夠使用。RemoteApp的特色在於透過遠端桌面連線的控制,把要顯示的資訊傳送到客戶本地端,由客戶本機端的Windows來負責產生畫面。
實作時是由伺服器端提供RemoteApp連線設定檔.rdp、或是包裝成.msi的安裝檔案,然後交給客戶端去做安裝與配置,例如放在桌面上提供使用者點選。這個RemoteApp連線設定檔本身已經包含了遠端伺服器的連線位置與登入資訊,以及要開啟的應用程式設定。客戶端只要開啟這個連線設定檔.rdp,等待連線,然後就會出現有如本機上運作的RemoteApp。
RemoteApp是伺服器類型的Windows才有提供的功能,但是Windows 7之類的個人電腦也可以作為RemoteApp伺服器。做法是使用RemoteApp Tool。
RemoteApp Tool適用於Windows XP SP3、Windows 7、Windows 8,幾乎目前常用的Windows都可以運作。
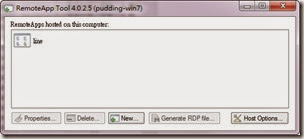
RemoteApp Tool不需要安裝,開啟之後就能夠設定要提供連線的應用程式。我自己馬上就建立了一個Line試試看。
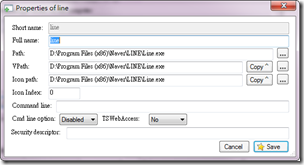
從Properties裡面可以設定應用程式的執行細節,最主要的是設定應用程式的執行路徑 Path。設定好RemoteApp之後,接著你可以使用Generate RDP file產生.rdp的遠端連線設定檔,再傳送到客戶端來連線。客戶端只要開啟.rdp,就會連線到這臺伺服器,然後開啟Line的應用程式。
雖然設定很簡單,但實際上運作時卻有許多問題。第一個是遠端連線速度不是很通順。開啟RemoteApp的時候,基本上就跟開啟遠端桌面連線一樣,但還要加入開啟應用程式的時間,因此連線時的時間會有不小的延遲。特別是當我們習慣開啟本地端的應用程式之後,再來跟RemoteApp開啟速度相比,就會覺得不甚理想。
然後是我使用Line的時候遇到了不少問題。也許是Line本身用了特殊的框架,導致開啟時不太順暢之外,一使用輸入法就會造成應用程式當機。
此外,這個RemoteApp Tool的另一個問題在於一次只能一個session連線。就跟一般Windows使用遠端桌面連線一樣,RemoteApp連線時就是佔了一個使用名額。當有別人開啟RemoteApp的時候,就算我來到伺服器的本地端,我看到的會是一個需要登入的畫面。而當我登入的時候,別人正在使用的RemoteApp則會被踢出去。
如果這些電腦都只有你個人使用的話,這倒沒什麼問題。但是在企業內希望使用RemoteApp共享安裝軟體的話,這問題就相當致命。由於Windows Server 2008等伺服器版本可以提供同一帳號多重遠端桌面,所以才能夠提供RemoteApp正常使用吧。不過如果Windows 7強制設定單一帳號多重登入的話,那也許也能夠提供一樣的功能也說不定。我稍微找了一下,不同的Windows版本有不同的設定方式:
但是因為RemoteApp感覺還是不太好用,所以我就嘗試找找看有沒有其他的替代方案,然後找到了Winflector。
RemoteApp替代方案:Winflector / RemoteApp Alternative Solution: Winflector
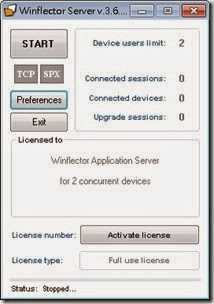
Winflector是一個類似RemoteApp的替代方案。免費版本可以提供兩個客戶端同時連線。
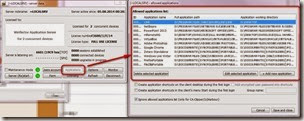
一開始一樣是先安裝Server版本,安裝完之後要重新開機。然後接著設定應用程式,這些設定類似RemoteApp。再來設定可以登入的使用者的帳號與密碼,這部分跟Windows的帳戶是分開獨立的。另外連接埠要修改掉被佔用的80,改為其他連接埠之後,才能正常連線。
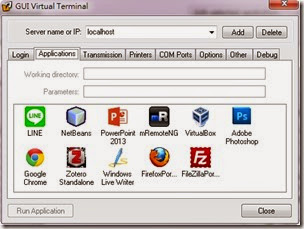
Winflector並不會產生像是RemoteApp這樣的連線設定檔,客戶端必須開啟Winflector客戶端程式,輸入伺服器端的IP連線資訊,接著就能夠在Applications看到伺服器端提供的應用程式,點選兩下就能夠直接開啟了。
開啟應用程式的速度不僅很快,而且輸入法、剪貼簿、視窗整合等操作都很流暢,彷彿真的就像是在本機端運作的程式一樣。而Winflector的連線也不會佔用遠端桌面連線的名額,不會把本機端使用者踢掉。
只是有幾點要注意的是事情:
- 應用程式仍然是在遠端伺服器運作,因此檔案存放的「桌面」是伺服器端的「桌面」,而不是客戶端的桌面。如果是RemoteApp的話,會直接開啟網路上的芳鄰來作為檔案交換的途徑,但是Winflector並沒有提供這種功能。
- 免費的Winfector只能提供兩個連線。
此外,Line開起來會一片空白,還是無法使用。
總而言之,Winfector意外地讓我很滿意,於是我最近會試著在桌機常駐Winfector的服務,讓筆電來連線使用看看。再試試看有沒有什麼問題。
為什麼團隊需要用RemoteApp? / Why Our Team Need RemoteApp?
個人使用的話,Winfector應該就能夠滿足需求了。就算是遠端檔案無法直接儲存在客戶端,也可以利用Dropbox來同步檔案。可是要在團隊裡面使用的話,這樣子的架構還不能算是完整。
為什麼團隊會需要使用RemoteApp?最大的原因仍然是因為軟體授權不足,或是軟體需要在高等級機器上運作,不方便安裝在筆電等效能較差的電腦上。
Citrix的XenApp / Citrix’s XenApp
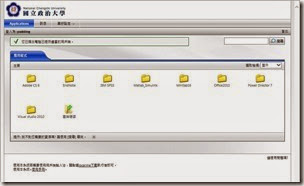
現在學校大多是使用Citrix的XenApp方案,這個方案其實也挺不錯的。它類似RemoteApp,但是運作RemoteApp的伺服器是以XenServer建構而成的Windows虛擬機器,而非實體的Windows。在每次要使用XenApp的時候,伺服器端會自動建立一個可以運作應用程式的虛擬機器,提供XenApp連線。然後在關閉XenApp之後把虛擬機器移除掉。因此每次開啟XenApp,看到的都會是預設軟體的樣子,使用者無法自訂軟體。
在檔案傳輸方面,XenApp會在連線時,把使用者本機端的硬碟作為網路上的芳鄰連線過去。使用XenApp開啟檔案時會看到磁碟機有幾個是網路上的硬碟,這才是本機端的硬碟。至於XenApp裡面看到的桌面跟C磁碟機都是虛擬機器本身的裝置,關閉XenApp之後就會消失。
在這樣的架構下,XenApp一樣有連線人數的限制,端看學校購買的授權數量,決定可以同時可以使用的連線人數。另一方面,XenApp的架構也是一個付費方案,所以就不是我的優先選擇。
Linux的X Window / Linux’s X Window
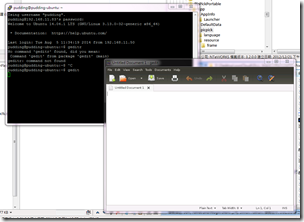
說到遠端桌面,大家是不是太專注在Windows上,而忘記了Linux本身就有強大的X Window呢?
其實X Window所提供的X11 Forwarding本身就能達到「只開啟應用程式」的效果。這篇SSH X11 Forwarding介紹的很詳細當然,鳥哥則是對X Window System則有較詳細的介紹鳥哥則是對X Window System則有較詳細的介紹。我也在Ubuntu上運作成功。做法是:
- 先在伺服器Ubuntu上安裝SSH:sudo apt-get install –y sshd
- 設定好Ubuntu的網路,確認客戶端Windows 7可以連線到Ubuntu
- 客戶端Windows 7上安裝Xming,要重開機
- 開啟PuTTY,設定連線到Ubuntu,啟用Enable X11 forwarding
- 登入SSH,進入命令列
- 以指令開啟要執行的應用程式,例如gedit
- 稍等片刻,客戶端Windows就會出現原本只能執行在Linux上的gedit
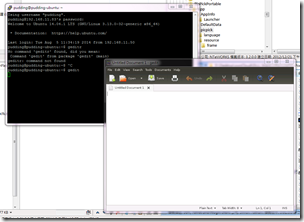
雖然步驟繁多,但是能在Windows開啟Linux的應用程式這點還是挺令人感到新奇的。不過實際上操作一下,發現問題有幾點:
- 剪貼簿運作正常,可以在Linux應用程式與Windows應用程式裡面複製貼上
- 無法使用Windows的輸入法,但可以複製貼上過去
- 檔案系統依然是Linux上的檔案系統
儘管如此,由於X Window並沒有限制連線數量,只要硬體效能足夠,應該可以提供大量使用者連線操作才是。
在Linux上運作Windows的程式 / Run Windows Application on Linux
如果要走X Window的方式提供遠端應用程式服務,那另一個問題在於,我們平常用的還是Windows的應用程式,而不是Linux的應用程式。
事實上,Linux有許多可以運作Windows應用程式的方法,以下兩個方案是最常見的做法:
這兩種方案都是使用轉換技術,講Windows的架構轉換成可以在Linux執行的環境,以在Linux開啟Windows的應用程式。雖然Wine是開放原始碼的軟體,但是使用上似乎有諸多問題。不同Wine版本、不同的Linux發佈版、不同的Windows應用程式版本之間對應會有許多問題。反觀CrossOver似乎評價就比較正面(也許是因為付費了,稱讚一下也好?)。
如果有這些軟體搭配的話,我在想可能的架構就可以變成:
- 在Linux伺服器上架設Wine環境
- 安裝Windows應用程式
- 客戶端上連線到Linux伺服器,開啟以Wine轉換的Windows應用程式
這樣子理想上就能達到不限人數的遠端應用程式的目標。不過我們還有其他問題還沒解決,一個是輸入法,另一個是檔案傳輸。輸入法的部分,這是另一個世界,我還得去研究看看;但是檔案傳輸的部分則比較簡單,我想可以用SFTP或是ownCloud來解決。
使用SSH交換遠端應用的檔案 / File Sharing with RemoteApp Server By SSH
最簡單的做法是使用SFTP連線到Linux伺服器。也就是說,連線到Linxu伺服器時,我們同時使用了三種服務:
- SSH command
- SSH X11 Forwarding
- SFTP
這三種都是走SSH連線,這樣做最單純。
小結:離實用仍有段距離 / Conclusion: Non-Ideal Solution
總歸上述,目前比較可能可以使用的方案有兩個:
- Winflector:Windows應用程式支援良好,但是限制人數2人
- Linux X Windows System:不限制人數,但是Windows應用程式支援堪慮
不論是那一種方案,其實都不甚理想。市面上也有許多付費的VDI應用方案,但我還是希望先能找到以自由軟體發佈的替代方案為主。
再等等看吧。
(more...)
Comments